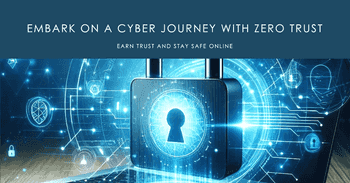
As developers, ensuring the security of our users' data is paramount, especially when dealing with sensitive information such as credentials. In Flutter, the default behavior of storing user input data as plain text in memory poses a significant security risk. In this blog post, we'll explore this problem and propose two solutions to mitigate it.
When a user interacts with a text field widget in Flutter, the entered data is stored as plain text in memory. This exposes sensitive information to potential threats, as memory dumps can be accessed by malicious actors, compromising user privacy and security.
To address this issue, we can create a custom text field widget that returns a list of strings instead of a single string. By storing user input as a list of strings, we obscure the data in memory, making it more challenging for attackers to extract sensitive information.
class SecureTextField extends StatefulWidget {
@override
_SecureTextFieldState createState() => _SecureTextFieldState();
}
class _SecureTextFieldState extends State<SecureTextField> {
List<String> _inputData = [];
@override
Widget build(BuildContext context) {
return TextField(
onChanged: (value) {
setState(() {
_inputData.add(value);
});
},
);
}
}
By utilising this custom text field widget, we enhance the security of user input data by storing it as a list of strings, mitigating the risk of plain text exposure in memory dumps.
Another approach to secure user input data is by implementing data obfuscation techniques. In this method, we replace entered characters with randomly generated characters, ensuring that the original input is not stored in memory.
String _obfuscateData(String input) {
String obfuscatedData = '';
for (int i = 0; i < input.length; i++) {
obfuscatedData += String.fromCharCode(Random().nextInt(255));
}
return obfuscatedData;
}
By replacing user input with randomly generated characters, we effectively mask the data stored in memory, making it extremely difficult for attackers to decipher sensitive information.
In this blog post, we've discussed the security implications of storing user input data as plain text in memory in Flutter applications. By implementing custom text field widgets and data obfuscation techniques, we can significantly enhance the security of user data and protect against potential threats.
As Flutter developers, it's our responsibility to prioritize the security and privacy of our users, and by adopting these solutions, we can build more resilient and secure applications.
Remember, security is not a one-time task but an ongoing process. Stay vigilant, keep learning, and always prioritise the protection of user data in your Flutter projects.